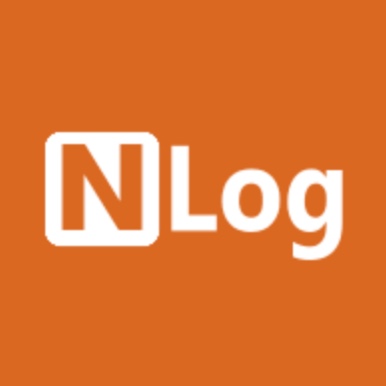
In NLog we can use the NLog.Web.AspNetCore package to write the status code with ${aspnet-response-statuscode}. We would like to extend that and also show the status description. In order to do this, we need to create a new layout renderer like this:
[LayoutRenderer("status-code-description")]
public class StatusCodeLayoutRenderer: AspNetResponseStatusCodeRenderer
{
protected override void Append(StringBuilder builder, LogEventInfo logEvent)
{
int statusCode = HttpContextAccessor.HttpContext.Response.StatusCode;
HttpStatusCode httpStatusCode = (HttpStatusCode)statusCode;
builder.Append($"{httpStatusCode}");
}
}
Now we need to add it to the nlog.config. Add the extensions section:
<extensions>
<add assembly="MyAssemblyName"/>
</extensions>
Then use it like any other renderer:
<attribute name="statusCode" layout="${aspnet-response-statuscode}"/>
<attribute name="statusDescription" layout="${status-code-description}"/>
There is also an option to set some context specific value which is valid for the current thread and available in the layout renderer, for example:
using var timestamp = ScopeContext.PushProperty("timestamp", HttpContext.Current.Timestamp);
Then use it withing the layout renderer:
if (ScopeContext.TryGetProperty("timestamp", out object timestamp))
{
DateTime dateTime = (DateTime)timestamp;
builder.Append($"{(DateTime.Now - dateTime).Milliseconds}");
}