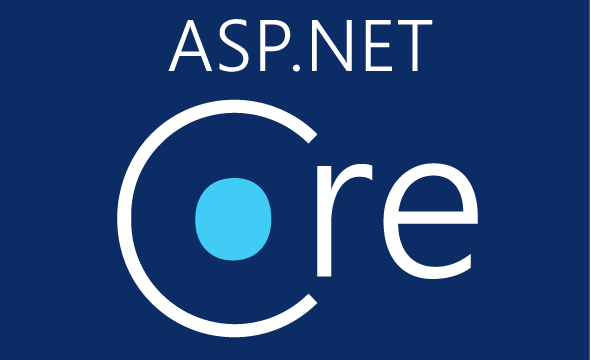
Use the page as a view model, to bind property use the [BindProperty] attribute.
In Razor HTML use the asp-for to do the binding
<input type='password' class="form-control" id='passcode' placeholder='passcode' asp-for="Password" />
Add action handlers such as OnGet or OnPost and make them return IActionResult.
public IActionResult OnPost()
{
return RedirectToPage("Content");
}
Here is an example of a conditional display
@if (!string.IsNullOrEmpty(Model.Error))
{
<div class="alert alert-danger mt-3">@Model.Error</div>
}
Example of injecting service defind in DI
@inject Microsoft.Extensions.Configuration.IConfiguration configuration
Loading partial views:
<partial name="~/Pages/SubViews/LogoImage.cshtml" model="configuration" />
When there are multiple forms - use asp-page-handler
<form action="post" asp-page-handler="edit">
<button>Edit</button>
<form>
then, add appropriate page handler:
public IActionResult OnPostEdit() {
}
The same works for Get requests, however, it won't work unless the HTTP request includes the "?handler=[handler_name]" query parameter. Make sure to add a hidden input field like the one below if you want the OnGetLogin handler to be invoked
<form method="get">
<input type="hidden" name="handler" value="login" />
<button type="submit" class="btn btn-primary">Login</button>
</form>
Query parameters
<a asp-route-a="a" asp-route-b="b">Click Here</a>
This translates to ?a=a&b=b
Bindable properties in the page model:
[BindProperty]
public string Field1 { get; set; }
[BindProperty]
public string Field2 { get; set; }
To get the values back use:
<input type="text" asp-for="MyProp" value="@Model.MyProp"/>
Validation text
<span asp-validation-for="Field1" class="text-danger"></span>
When using a textarea, the asp-for does not work, so replace it with this code:
<textarea class="form-control" readonly name="@Html.NameFor(m => m.MyProp)">@Model.MyProp</textarea>
Using values from a query
[BindProperty(SupportsGet = true)]
[FromQuery(Name = "Id")]
public sint? Id { get; set; }
Return a file
private IActionResult Deliver(MemoryStream memoryStream, string mimetype)
{
memoryStream.Seek(0, SeekOrigin.Begin);
return new FileStreamResult(memoryStream, mimetype)
{
FileDownloadName = "report.pdf"
};
}
Create an Excel report
XLWorkbook wb = new();
IXLWorksheet ws = wb.AddWorksheet();
ws.Cell(1, 1).SetValue("Field one");
ws.Cell(1, 2).SetValue("Field two");
ws.Cell(1, 1).SetValue("Field one value");
ws.Cell(1, 2).SetValue("Field two value");
ws.Columns().AdjustToContents();
MemoryStream memoryStream = new();
wb.SaveAs(memoryStream);
memoryStream.Seek(0, SeekOrigin.Begin);
return Deliver(memoryStream, "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet");