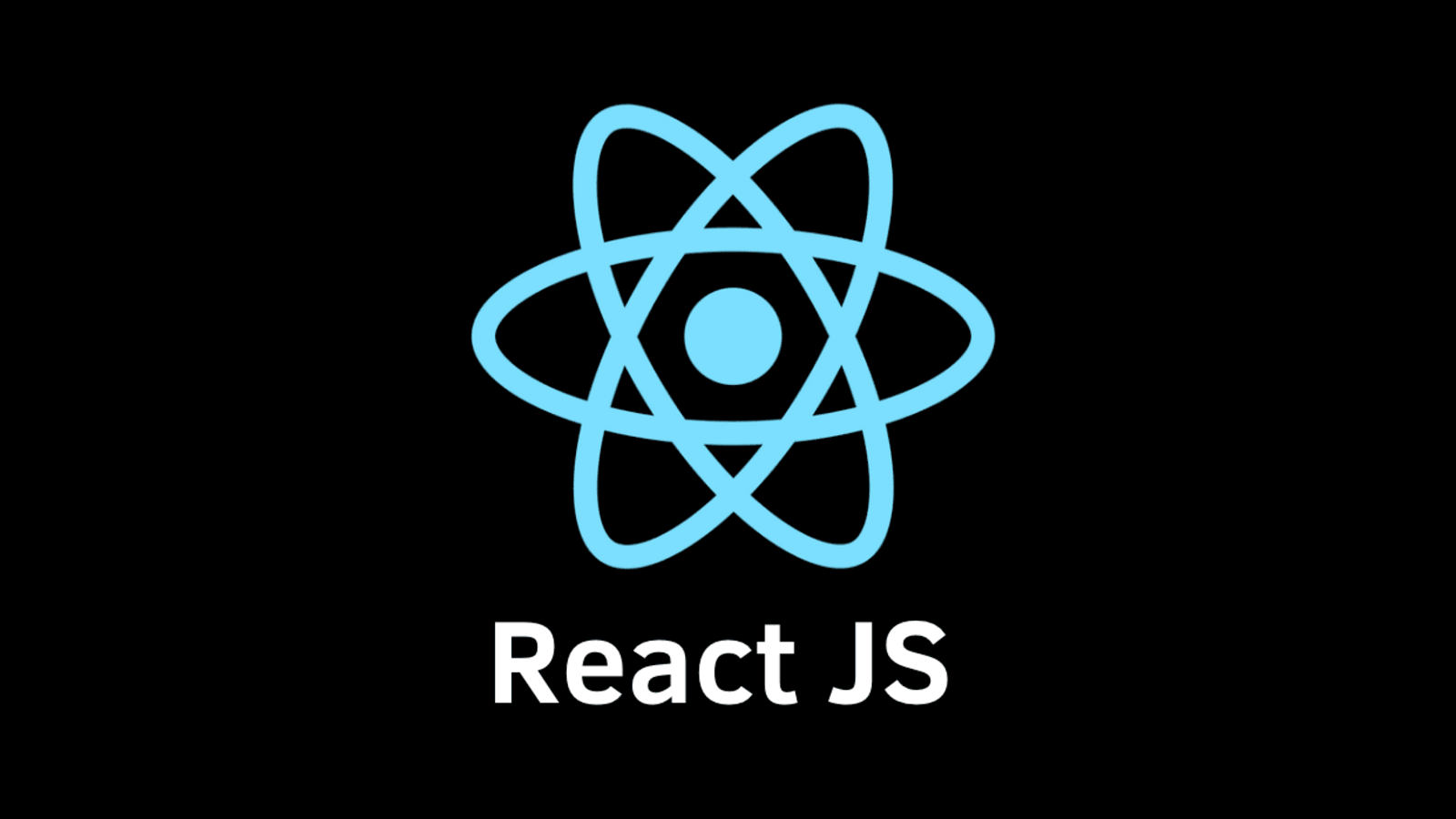
Install the "react-paginate" library by:
npm install --save "react-paginate"
Set pagination state:
const itemsPerPage = 10;
const [pageCount, setPageCount] = useState(1);
const [itemOffset, setItemOffset] = useState(0);
const [totalCount, setTotalCount] = useState(0);
const [currentPage, setCurrentPage] = useState(0);
const [queryParams, setQueryParams] = useState({ idnumber: "", phone: "" });
const [data, setData] = useState([]);
const addQueryParam = (key, value) => {
const url = new URL(window.location.href);
url.searchParams.set(key, value);
window.history.pushState({}, '', url.toString());
};
const getQueryParam = (key) => {
const url = new URL(window.location.href);
return url.searchParams.get(key) || '';
};
async function search(e) {
if (e) {
/* This implies the call was done by clicking the "search" button and not by clicking on the pagination bar */
e.preventDefault();
setItemOffset(0);
setCurrentPage(0);
}
const endOffset = itemOffset + itemsPerPage;
const count = endOffset - itemOffset;
const result = await axios.get(`${process.env.PUBLIC_URL}/server/customers/list` +
`?offset=${itemOffset}&count=${count}` +
`&idnumber=${queryParams.idnumber}&phone=${queryParams.phone}`);
setTotalCount(+result.headers["x-total-count"]);
setData(result.data);
setPageCount(Math.ceil(+result.headers["x-total-count"] / itemsPerPage));
addQueryParam("offset", itemOffset);
addQueryParam("idnumber", queryParams.idnumber);
addQueryParam("phone", queryParams.phone);
}
const handlePageClick = (event) => {
setCurrentPage(event.selected);
const newOffset = (event.selected * itemsPerPage) % totalCount;
setItemOffset(newOffset);
search();
};
Render the pagination bar, this example uses bootstrap 5 for styling.
<nav>
<ReactPaginate
breakLabel="..."
nextLabel="next >"
onPageChange={handlePageClick}
pageRangeDisplayed={5}
pageCount={pageCount}
previousLabel="< previous"
renderOnZeroPageCount={null}
containerClassName="pagination"
pageClassName="page-item"
previousClassName="page-item"
previousLinkClassName="page-link"
nextClassName="page-item"
nextLinkClassName="page-link"
pageLinkClassName="page-link"
activeClassName="active"
forcePage={currentPage}
/>
</nav>
Tags
React