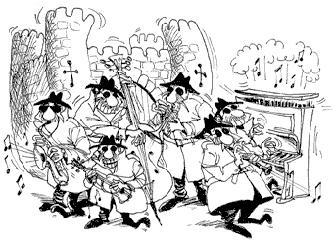
The AIA extension can hold a URL to an OCSP server or to the certificate of the issuer. Here is an example of building the chain by parsing this extension with the bouncycastle library
private static List<string> GetUrls(Org.BouncyCastle.X509.X509Certificate cert)
{
List<string> caUrls = new List<string>();
byte[] encodedAia = cert.GetExtensionValue(X509Extensions.AuthorityInfoAccess).GetOctets();
AuthorityInformationAccess instance = AuthorityInformationAccess.GetInstance(Asn1Object.FromByteArray(encodedAia));
foreach (AccessDescription accessDescription in instance.GetAccessDescriptions())
{
if (accessDescription.AccessMethod.Id == X509ObjectIdentifiers.IdADCAIssuers.Id)
{
string url = accessDescription.AccessLocation.Name.ToString();
caUrls.Add(url);
}
}
return caUrls;
}
private static bool GetChain(byte[] certBuffer, List<Org.BouncyCastle.X509.X509Certificate> chain)
{
Org.BouncyCastle.X509.X509Certificate caCert;
X509CertificateParser parser = new X509CertificateParser();
List<string> caUrls = GetUrls(parser.ReadCertificate(certBuffer));
foreach (string caUrl in caUrls)
{
try
{
WebClient webClient = new WebClient();
byte[] caCertBuffer = webClient.DownloadData(caUrl);
caCert = parser.ReadCertificate(caCertBuffer);
chain.Add(caCert);
if (caCert.IssuerDN.Equivalent(caCert.SubjectDN))
{
return true;
}
return GetChain(caCertBuffer, chain);
}
catch
{
}
}
return false;
}