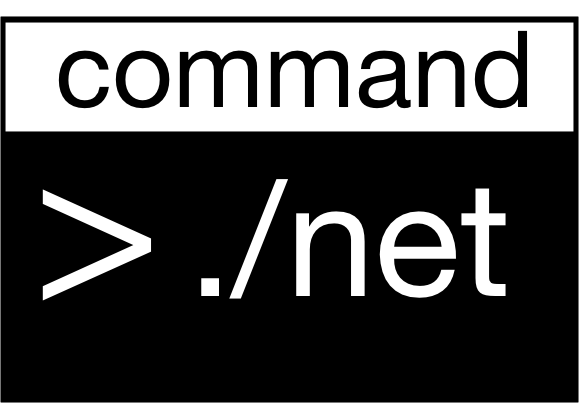
In .NET Framework
Using the ServiceBase class:
static void Main(string[] args)
{
ServiceBase[] ServicesToRun;
ServicesToRun = new ServiceBase[]
{
new MyNewService(args)
};
ServiceBase.Run(ServicesToRun);
}
Then, to install it use the installutil.exe utility:
installutil.exe [service.exe]
To uninstall, run it with the /u switch
In .Net core
Use nuget to install the
Microsoft.Extensions.Hosting.WindowsServices
package.
Create a worker service:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.UseWindowsService()
.ConfigureServices(hostContext, services =>
{
services.AddHostedService<Worker>();
});
}
public class Worker : BackgroundService
{
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
// do stuff here
}
}
Then install it with SC create
sc.exe create MyAppService binpath= c:\service.exe start= auto
or delete with
sc delete
Any windows executable:
which is especially useful for Java
Java
I found this tool:
http://winrun4j.sourceforge.net/
as the best choice if NSSM is not an option