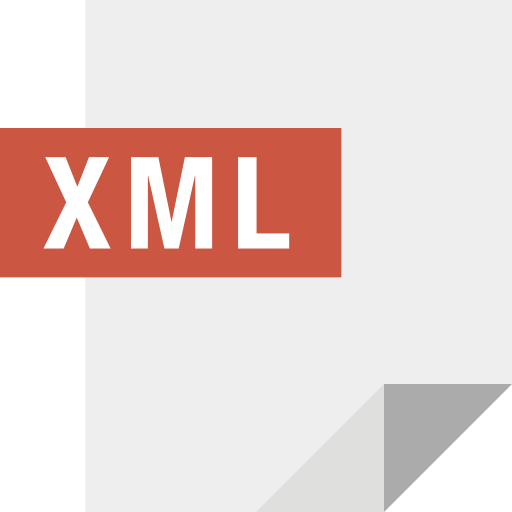
This article shows how to deserialize an object stored in an XML format
Assume we have the following .NET class
public class MyItem
{
public string ItemProp {get;set;}
}
public class MyData
{
public string MyProp1 {get;set;}
public int MyProp2 {get;set;}
public List<MyItem> Items {get;set;}
}
The corresponding XML should be of this form:
<MyData>
<MyProp1>prop1</MyProp1>
<MyProp2>2</MyProp2>
<Items>
<MyItem>
<ItemProp>a</ItemProp>
</MyItem>
<MyItem>
<ItemProp>b</ItemProp>
</MyItem>
</Items>
</MyData>
We need to decorate the .NET class with the following attributes:
public class MyItem
{
[XmlElement("ItemProp")]
public string ItemProp {get;set;}
}
[XmlRoot("MyData")]
public class MyData
{
[XmlElement("MyProp1")]
public string MyProp1 {get;set;}
[XmlElement("MyProp2")]
public int MyProp2 {get;set;}
[XmlElement("Items")]
public List<MyItem> Items {get;set;}
}
To deserialize use this:
XmlSerializer serializer = new XmlSerializer(typeof(MyData));
using (TextReader reader = new StringReader(...))
{
MyData result = (MyData) serializer.Deserialize(reader);
}