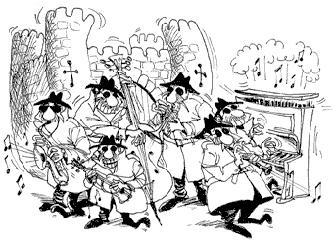
First we generate an RSA key pair, then we add the certificate attributes and finally create a signature factory (instead of the deprecated way of using the private key directly).
public void GenerateCertificateWithKey(string subjectDn)
{
RsaKeyPairGenerator rsaKeyGenerator = new ();
KeyGenerationParameters keyGenerationParameters = new (new SecureRandom(), 2048);
rsaKeyGenerator.Init (keyGenerationParameters);
AsymmetricCipherKeyPair pair = rsaKeyGenerator.GenerateKeyPair();
X509Name subjectName = new (subjectDn);
X509V3CertificateGenerator certGenerator = new();
certGenerator.SetSerialNumber(BigInteger.ValueOf(DateTime.Now.Ticks));
certGenerator.SetIssuerDN(subjectName);
certGenerator.SetNotBefore(DateTime.UtcNow);
certGenerator.SetNotAfter(DateTime.UtcNow.AddYears(4));
certGenerator.SetSubjectDN(subjectName);
certGenerator.SetPublicKey(pair.Public);
certGenerator.AddExtension(X509Extensions.BasicConstraints, true,
new BasicConstraints(false));
certGenerator.AddExtension(X509Extensions.KeyUsage, true, new KeyUsage(
KeyUsage.NonRepudiation));
ISignatureFactory signatureFactory = new Asn1SignatureFactory("SHA512WITHRSA", pair.Private, new SecureRandom());
var cert = certGenerator.Generate(signatureFactory);
}
Tags
bouncycastle