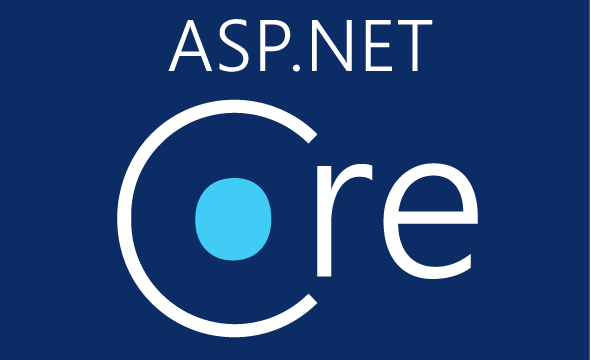
Creating JWT Token
First we need to have some tool or code that to let us test JWT tokens.
Our JWT tokens will be signed with an RSA key.
We can generate a certificate with a private key.
Create a .NET core application and add this package:
System.IdentityModel.Tokens.Jwt
Then add this code:
var tokenHandler = new JwtSecurityTokenHandler();
var signingKey = new X509SecurityKey(SigningCertificate);
var tokenDescriptor = new SecurityTokenDescriptor
{
Subject = new ClaimsIdentity(new Claim[]
{
new Claim(ClaimTypes.Name, Sub),
/*new Claim(ClaimTypes.Role, "Author"),*/
}),
Claims = new Dictionary<string, object>() {
[JwtRegisteredClaimNames.Sub] = Sub
},
Expires = Exp,
SigningCredentials = new SigningCredentials(signingKey, SecurityAlgorithms.RsaSha256Signature)
};
if (!string.IsNullOrEmpty(Aud))
tokenDescriptor.Audience = Aud;
var token = tokenHandler.CreateToken(tokenDescriptor);
string result = tokenHandler.WriteToken(token);
The SigningCertificate should be an X509Certificate2 instance built from the certificate created with the key explorer tool (or something else).
Enforcing JWT Bearer authentication
In the ASP.NET core application we should register the authentication and authorization services:
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
//options.Authority = Configuration["Authorization:Issuer"];
options.Audience = Configuration["Authentication:Audience"];
options.IncludeErrorDetails = true;
var jwtSignerCertPath = Configuration["Authentication:JwtSigner"];
if (File.Exists(jwtSignerCertPath))
{
byte[] buffer = File.ReadAllBytes(jwtSignerCertPath);
var x509Cert = new X509Certificate2(buffer);
SecurityKey key = new X509SecurityKey(x509Cert);
options.TokenValidationParameters.IssuerSigningKey = key;
}
options.TokenValidationParameters.ValidateAudience = true;
options.TokenValidationParameters.ValidateLifetime = true;
options.TokenValidationParameters.ValidateIssuer = true;
options.TokenValidationParameters.ValidateIssuer = false;
options.TokenValidationParameters.ValidateActor = false;
//options.TokenValidationParameters.NameClaimType = JwtRegisteredClaimNames.Sub;
/* By default the User.Identity.Name will be inferred from the "name" claim of the JWT */
});
services.AddAuthorization(options =>
{
options.AddPolicy("Default", policy =>
policy.RequireAssertion(context =>
context.User != null
/*context.User.HasClaim(claim =>
claim.Type == "aud" && claim.Value == Configuration["Authentication:Audience"]
)
*/
)) ;
});
Also we need to add authentication and authorization middleware:
app.UseAuthentication();
app.UseAuthorization();
Tags
ASP .NET core