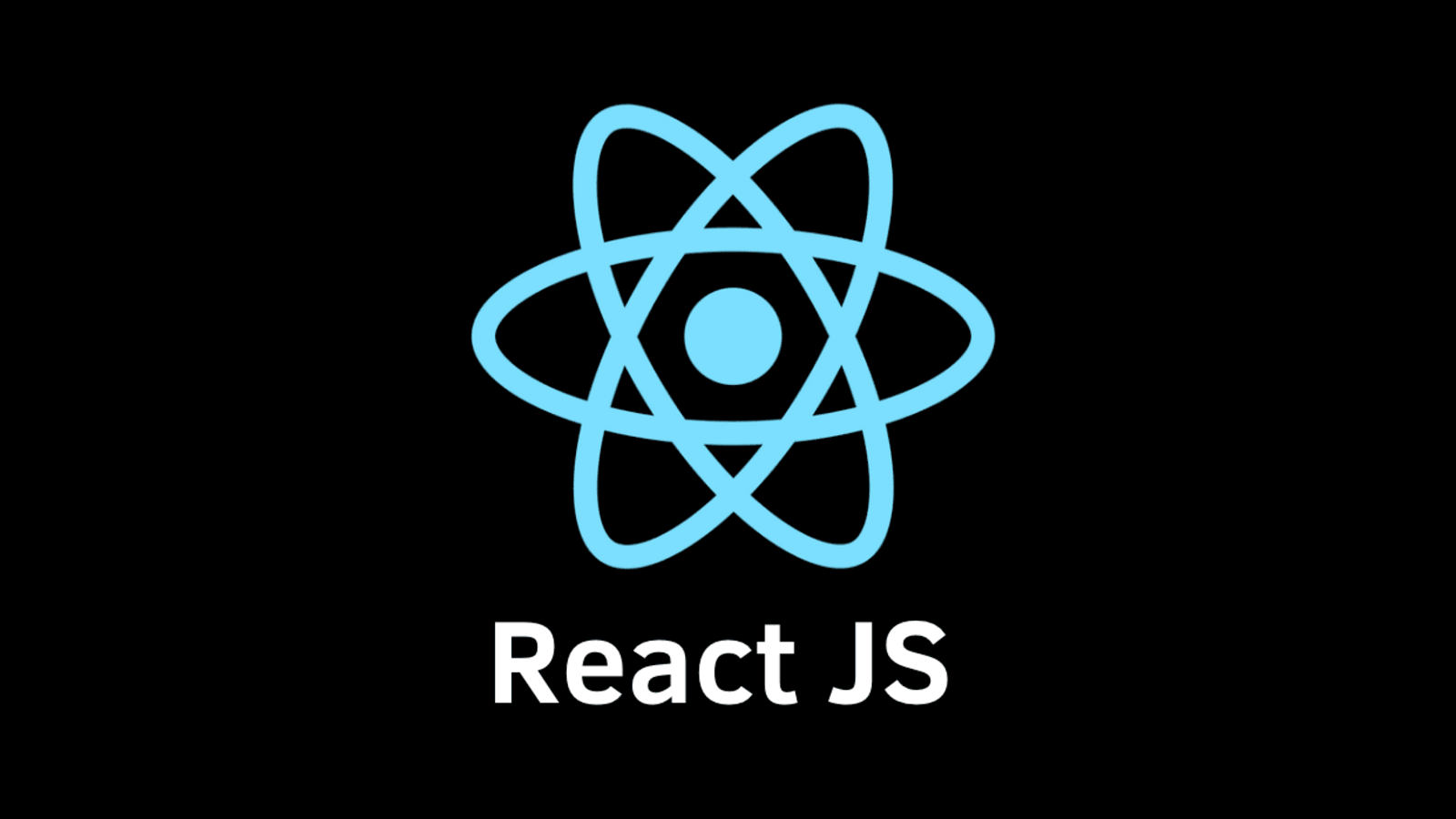
React Routing
To use routing, install the following package:
npm install react-router-dom
Wrap your main component <BrowserRouter> or <HashRouter>
<BrowserRouter>
<App />
</BrowserRouter>
Then use the Routes component to display the current page.
<Routes>
<Route path="/first" element={<FirstPage/>} />
<Route path="/second/:id" element={<SecondPage/> } />
</Routes>
To create a redirect use the Navigate component like this:
<Route path="/" element={<Navigate replace to="/first" />}/>
To navigate use the <Link to="first">First</Link> component or write directly <a href="/first">First</a>
Here is a full example:
class FirstPage extends Component {
render() {
return (
<div>First Page</div>
);
}
}
class SecondPage extends Component {
render() {
return (
<div>Second Page</div>
);
}
}
function App() {
return (
<div className="App">
<div>
<Link to="/first">Open First Page</Link>
<br/>
<Link to="/second">Open Second Page</Link>
</div>
<Routes>
<Route path="" element="<Navigate replace to="/first" />" />
<Route path="/first" element={<FirstPage />} />
<Route path="/second" element={<SecondPage />} />
</Routes>
</div>
);
}
To navigate programmatically, use this hook
const navigation = useNavigation();
Navigate
navigate("/first");
Navigate with parameters
navigate("/second", { id: 123});
Go back with (equivalent to browser go back):
navigate(-1)
Read parameters with:
let { id } = useParams();
Add or get search query parameters without reloading the page:
const addQueryParam = (key, value) => {
const url = new URL(window.location.href);
url.searchParams.set(key, value);
window.history.pushState({}, '', url.toString());
};
const getQueryParam = (key) => {
const url = new URL(window.location.href);
return url.searchParams.get(key) || '';
};
Previous Part