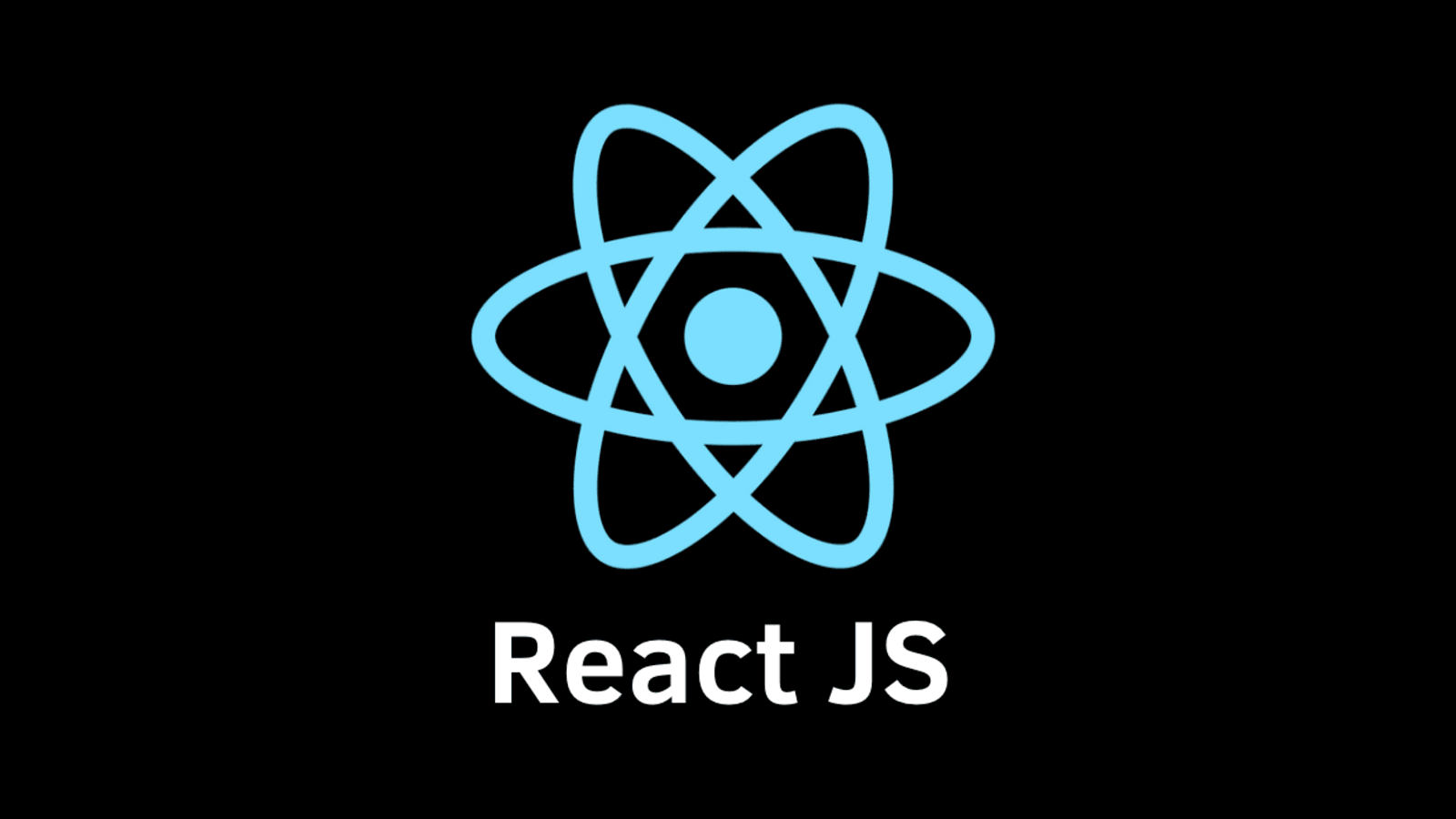
Installing React
Install react scripts (create-react-app)
npm install react-scripts@latest
Create new application from some template or use a default.
npx create-react-app myapp --template cra-template-tailwindcss
To find more templates, search in npm for "cra-template-*"
Alternative way to create a react app is to use the vite.js which is more advanced with various advantages such as much faster build time, run:
npm create vite@latest
Components
The basic building block in React is a component.
Create a function component like this:
function Header() {
return (
<div>Something</div>
)
}
Then, use this component as an element in a JSX.
<Header/>
Maintain a state like this:
const [someData, setSomeData] = useState({ "field1": "value1", "field2": "value2"})
To get the current state use "someData", to set a new state, e.g. update part of it, use the spread operator:
setSomeData({...someData, "field1": "value11111"})
comments in the JSX should be enclosed in {* *}
Interaction between components
A parent component may pass data to a child component as a property:
<Child myprop={parentdata}/>
Then, in the child component, get access to this data like this
function Child({myprop}) {
...
}
The child component may receive a callback function
function Child({onSomeEvt}) {
...
onSomeEvt();
}
Component lifecycle events
Do it in React with the useEffect hook like this
function MyComp {
useEffect(() => {
... startup
}, []);
...
}
We can also run effect on specific variable change
useEffect(() => {
... hanfle change
}, [myprop]);
Add a cleanup handler
useEffect(() => {
return function clean() {
... some cleanup code
};
}, []);