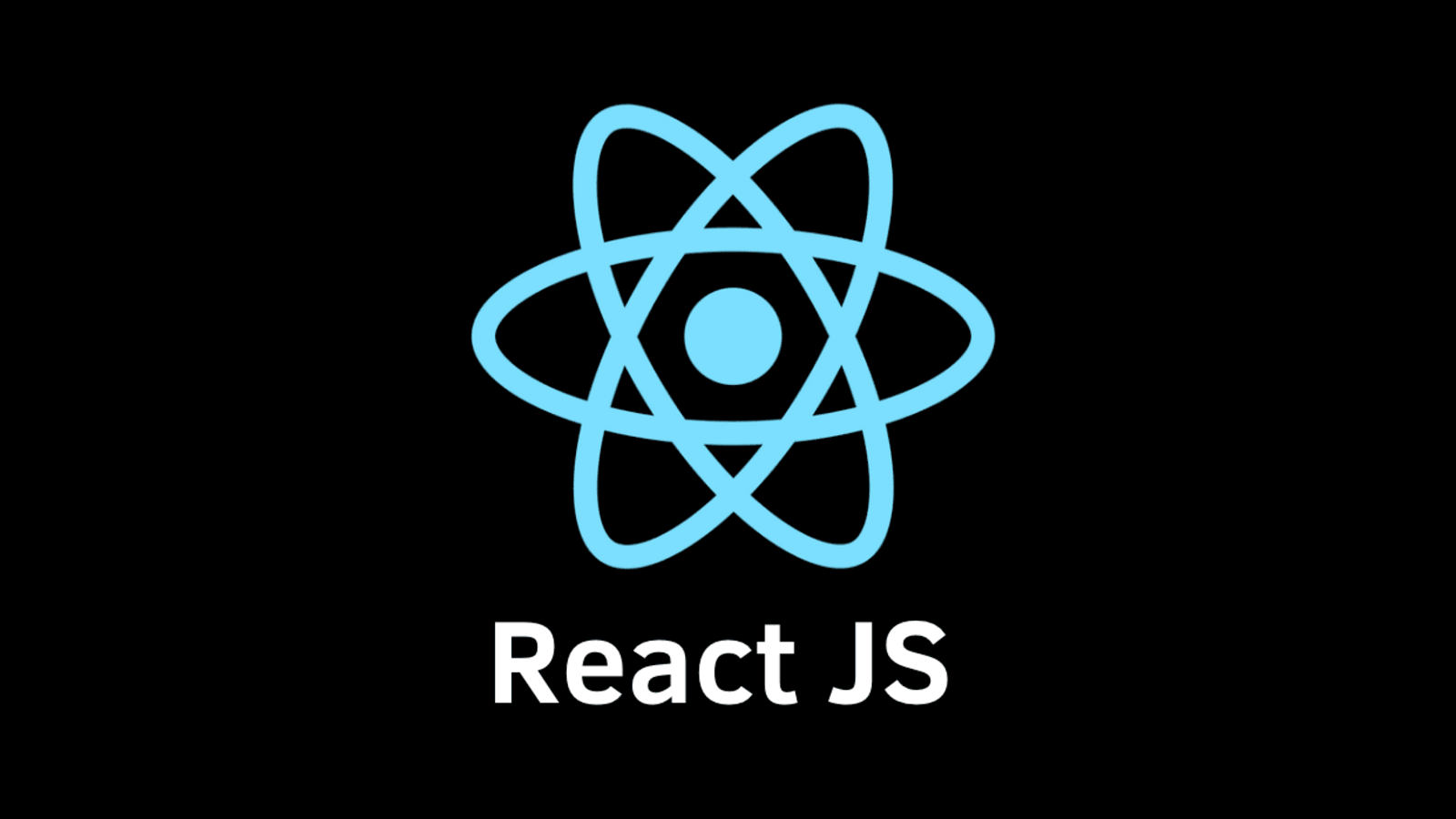
We want a simple way to display toasts in a specific position on the app for notifications from various components. For the simplicity we will display only one toast at a time. With react it is better to use the react-bootstrap library
The code below creates a context that will pass a toast update method to all child components of the app.
import { useState } from "react";
import { createContext } from "react";
import { Toast, ToastBody, ToastContainer } from "react-bootstrap";
export const ToastsContext = createContext();
const Toasts = ({children}) => {
const [toastData, setToastData] = useState({ variant: '', text: ''});
const [show, setShow] = useState(false);
function setToast(variant, text) {
setToastData({variant, text});
setShow(true);
}
return (
<ToastsContext.Provider value={setToast}>
<ToastContainer position="top-end">
<Toast bg={toastData.variant} className="mt-1 me-1" delay={3000} autohide show={show} onClose={() => setShow(false)}>
<ToastBody className="text-white">
{toastData.text}
</ToastBody>
</Toast>
</ToastContainer>
{children}
</ToastsContext.Provider>
);
}
export default Toasts;
Now, we need to create a custom hook that can be consumed by the child components in order to show the toast
import { useContext } from "react";
import { ToastsContext } from "./toasts";
function useToast() {
const setToast = useContext(ToastsContext);
function showSuccess(text) {
setToast('success', text);
}
function showError(text) {
setToast('danger', text);
}
return [showSuccess, showError];
}
export default useToast;
The actual call for a toast is as following:
...
const [showSuccess, showError] = useToast();
...
showSuccess('Saved successfully');
...
showError('Internal error');
...
Tags
React