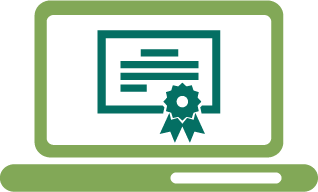
Here is an example of getting the directory address from the X509Certificate2 class using the System.Formats.Asn1 library (install from nuget)
X509Certificate2 cert = ...
if (cert.Extensions.OfType<X509Extension>().FirstOrDefault(ex => ex.Oid.Value == "2.5.29.17") is X509Extension sanExtension)
{
AsnReader asnReader = new AsnReader(sanExtension.RawData, AsnEncodingRules.DER);
asnReader = asnReader
.ReadSequence();
//parse general names
while (asnReader.HasData)
{
Asn1Tag tag = asnReader.PeekTag();
if (tag.TagValue == 4)
{
asnReader = asnReader.ReadSetOf(tag);
byte[] dnBytes = asnReader.ReadEncodedValue().ToArray();
AsnEncodedData givenNameData = new AsnEncodedData(new Oid("2.5.4.42"), dnBytes);
string givenName = givenNameData.Format(false);
AsnEncodedData surnameData = new AsnEncodedData(new Oid("2.5.4.4"), dnBytes);
string surname = givenNameData.Format(false);
break;
}
asnReader.ReadEncodedValue();
}
}
For .NET 7 or 8, there is a class that can easily parse the alternative name extension, so the purpose of the article is to demonstrate how to implement this with .NET framework or for older .NET versions
Previously I've explained how to implement this with bouncy castle library: https://alexrait.blogspot.com/2023/10/parse-subject-alternative-directory.html
Tags
Certificates