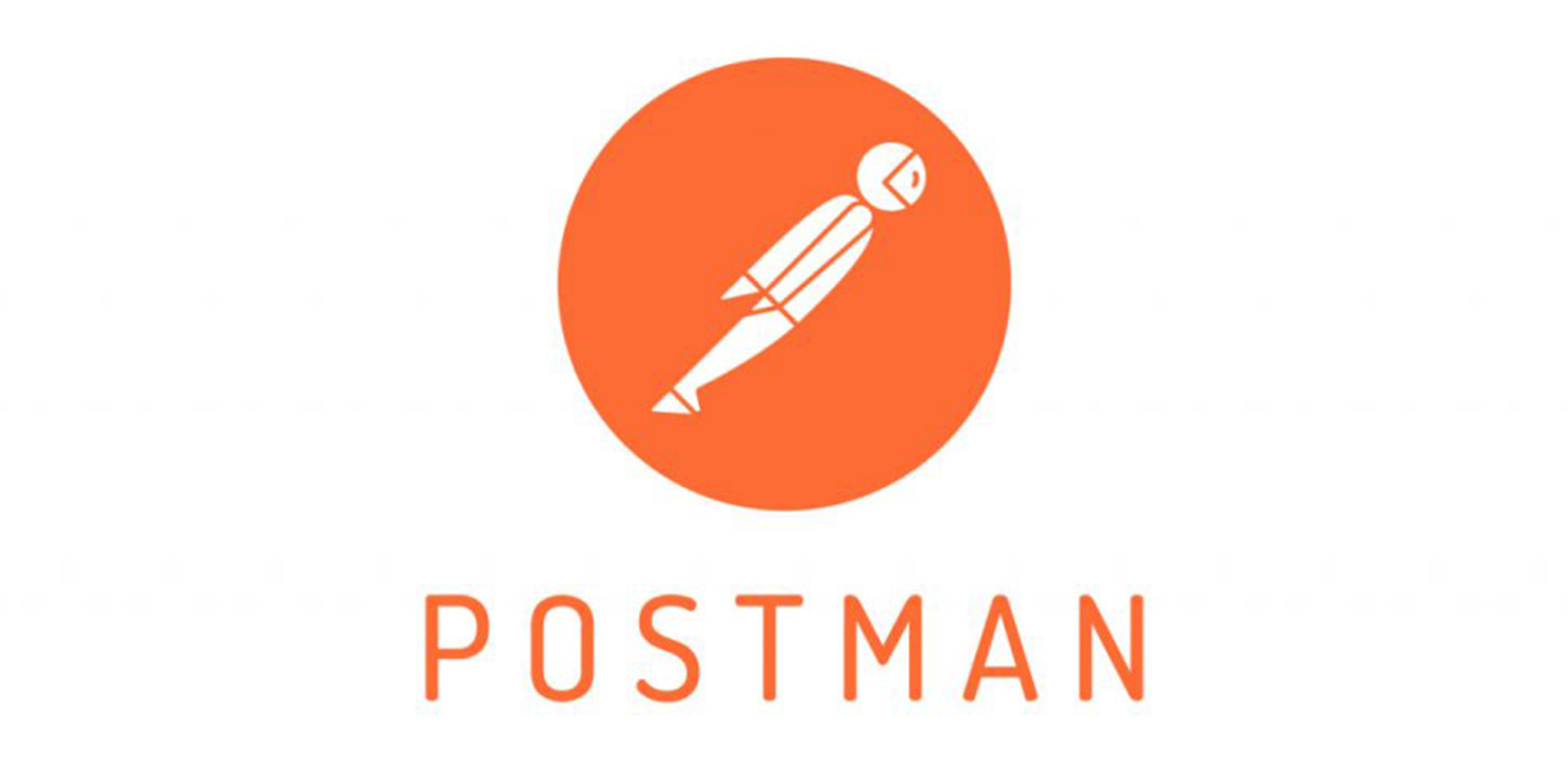
Compute a SHA256 hash, then encode it as base64 string
var base64 = CryptoJS.SHA256("test").toString(CryptoJS.enc.Base64);
console.log(base64);
Save a response as collection variable
var json = JSON.parse(responseBody);
pm.collectionVariables.set("SomeProp", json.SomeProp);
Create a GUID in the pre request script
var uuid = require('uuid');
var guid = uuid.v4();
Generate random string of some length
function randomString(minValue, maxValue, dataSet = 'abcdefghijklmnopqrstuvwxyz0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ') {
if (!minValue) {
minValue = 20;
maxValue = 20;
}
if (!maxValue) {
maxValue = minValue;
}
let length = _.random(minValue, maxValue),
randomString = "";
for (let i = 0; i < length; i++)
randomString += dataSet.charAt(Math.floor(Math.random() * dataSet.length));
return randomString;
}
pm.collectionVariables.set("randomString", randomString());