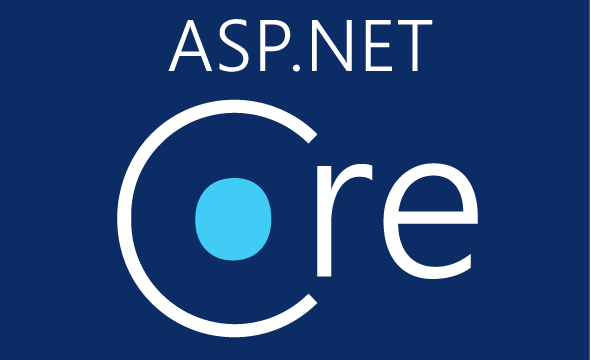
To add swagger + swagger UI follow these steps:
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo
{
Version = "v1",
Title = "This is a title",
Description = "This is a description",
TermsOfService = new Uri("https://someurl"),
Contact = new OpenApiContact
{
Name = "Some name",
Email = string.Empty,
Url = new Uri("https://someurl"),
},
License = new OpenApiLicense
{
Name = "Some license",
Url = new Uri("https://someurl"),
}
});
var xmlFile = $"{Assembly.GetExecutingAssembly().GetName().Name}.xml";
var xmlPath = Path.Combine(AppContext.BaseDirectory, xmlFile);
c.IncludeXmlComments(xmlFile );
});
Add packages from NuGet:
Swashbuckle.AspNetCore
Swashbuckle.AspNetCore.Annotations
Add swagger middleware:
app.UseSwagger();
app.UseSwaggerUI(
c =>
{
c.SwaggerEndpoint("swagger/v1/swagger.json", "My API V1");
c.RoutePrefix = "";
});
If the application is not a root website but rather a web application which is a subsite, this statement ensures the right request path is passed to the .NET core application.
To describe the swagger actions we need to use XML comments, edit the .proj file and add this:
<PropertyGroup>
<GenerateDocumentationFile>true</GenerateDocumentationFile>
<NoWarn>$(NoWarn);1591</NoWarn>
</PropertyGroup>
Then use comments like this:
/// <summary>
/// Doing some important action
/// </summary>
/// <param name="param1">The data</param>
/// <remarks>
/// Sample request:
///
/// POST /action
/// {
/// "Field1": "Value1",
/// "Field2": "Value2"
/// }
///
/// </remarks>
/// <returns>On success, returns the id number of the new entity</returns>
Also, explain the response options:
[SwaggerResponse(200, @"On Success", typeof(Response1))]
[SwaggerResponse(400, @"On Bad Request", typeof(Response2))]
[SwaggerResponse(500)]
Use this filter to expose enum values correctly:
public class EnumSchemaFilter : ISchemaFilter
{
public void Apply(OpenApiSchema model, SchemaFilterContext context)
{
if (context.Type.IsEnum)
{
model.Enum.Clear();
Enum.GetNames(context.Type)
.ToList()
.ForEach(name => model.Enum.Add(
new OpenApiString($"{name}({Convert.ToInt64(Enum.Parse(context.Type, name))})")));
}
}
}
Add the filer at AddSwaggerGen callback:
c.SchemaFilter<EnumSchemaFilter>();
Tags
ASP .NET core